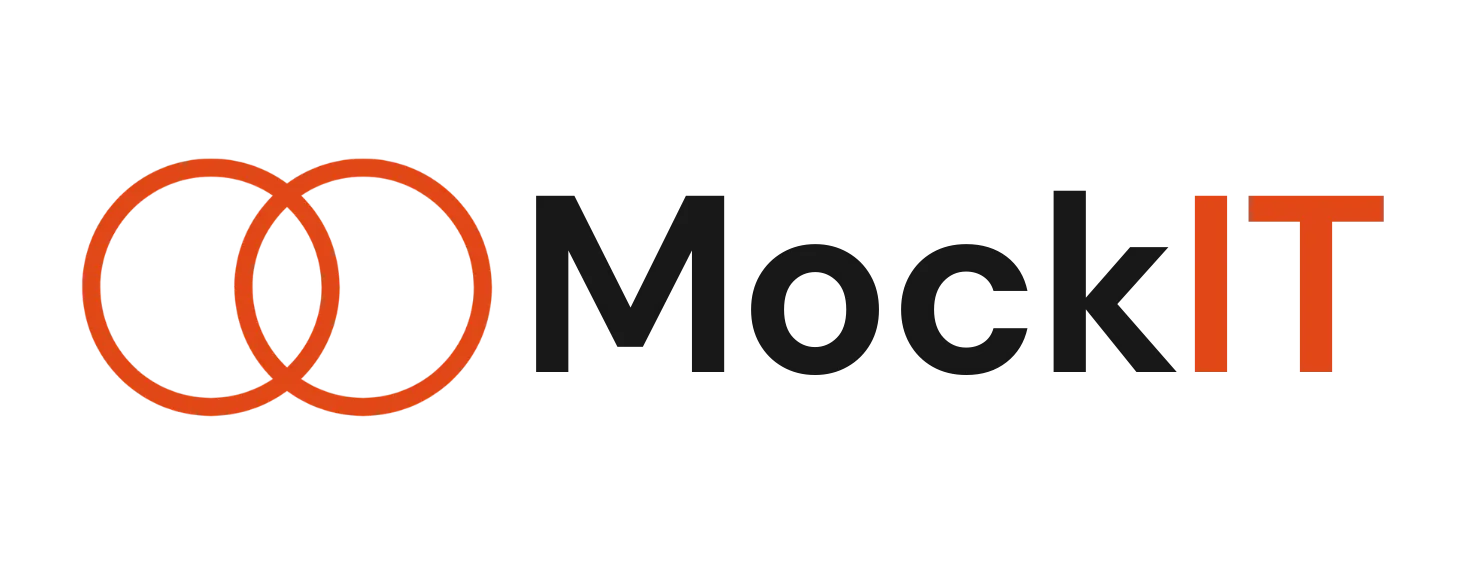
Do you get the feeling that no one is reading your CV? Not getting invitations to recruitment interviews?
Test your technical skills and let MockIT help find you a job in IT!
React Job Interview - Typical Recruitment Questions
Are you preparing for technical interviews for a React Frontend Developer position? Or maybe you want to refresh your knowledge on this topic? In this article, you will find several basic concepts without which working with React would be impossible!
Intro
React, being one of the most popular JavaScript libraries, has become an indispensable tool in the arsenal of a modern programmer building web applications. Its flexibility and performance attract millions of developers who use its capabilities daily. However, despite its widespread use, candidates often encounter difficulties related to a deep understanding of its basic mechanisms.
Preparing for job interviews requires not only technical knowledge but also the ability to apply this knowledge to practical tasks. This article aims to help candidates understand five key questions that may arise during a job interview, to better prepare for answering them, significantly increasing their chances of success.
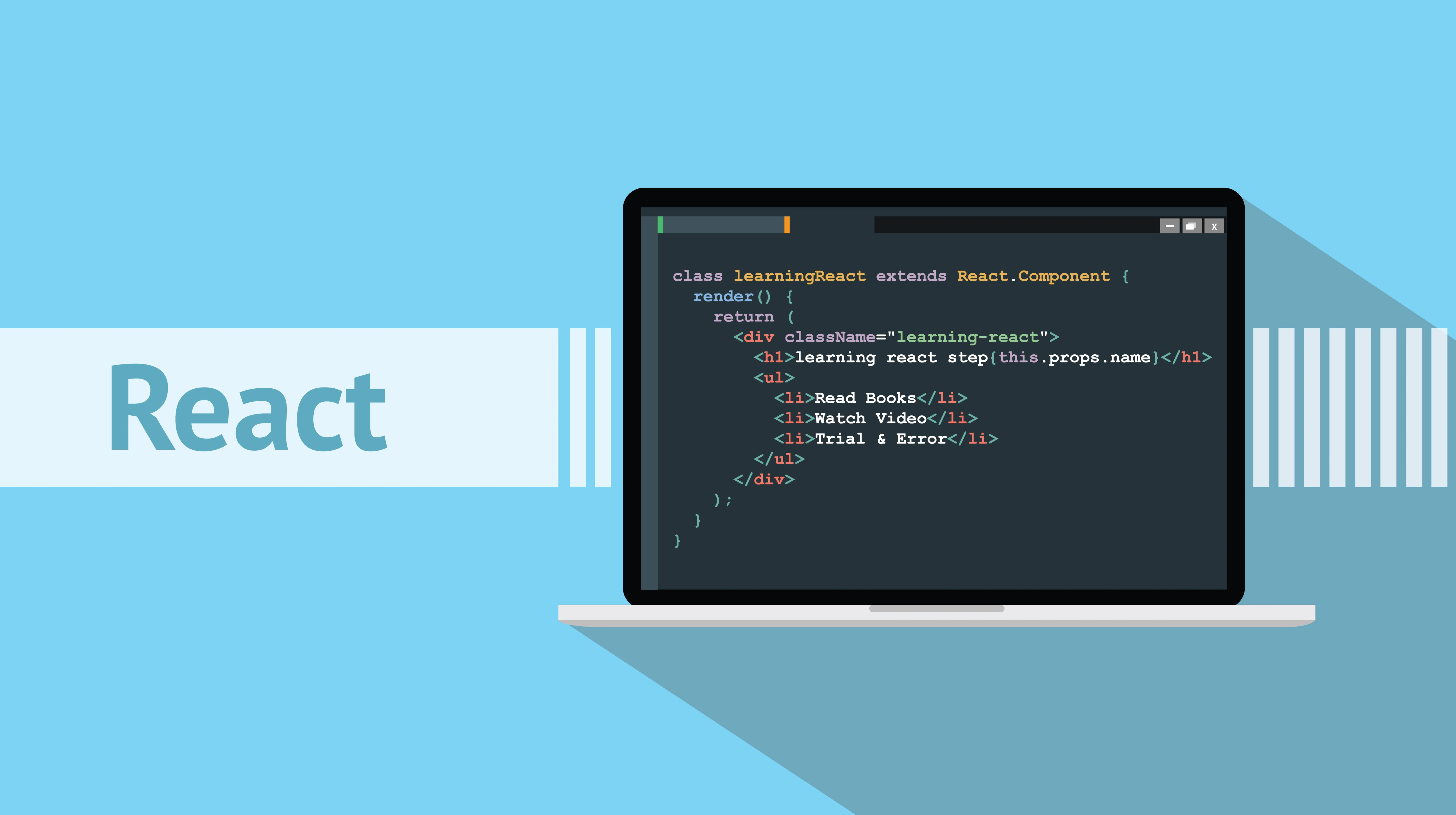
Question 1: What is JSX and what significance does it have in React?
JSX, which stands for JavaScript XML, is a syntactic extension for JavaScript that allows developers to write HTML elements directly in JavaScript code. Thanks to this, instead of using the React.createElement method for manual creation of DOM elements, programmers can use tags similar to HTML, which significantly simplifies the code and makes it easier to maintain.
const element = <h1>Hello, world!</h1>;
In the above example, JSX is transpiled to the function:
React.createElement('h1', null, 'Hello, world!');
which allows React to process and render user interface elements. Using JSX not only facilitates the creation of components with a more understandable syntax but also increases the library's performance, enabling automatic optimization of the process of creating and updating the DOM. Additionally, support for JavaScript expressions in JSX code allows developers to easily integrate application logic directly into the component structure, which is crucial for building dynamic user interfaces.
Question 2: What is the Virtual DOM in React and how does it impact application performance?
During a job interview for a React developer position, a question about the Virtual DOM allows technical recruiters to assess how well the candidate understands the key optimization mechanisms of this library. The Virtual DOM is a concept that significantly increases application performance by minimizing direct interactions with the browser's real DOM (Document Object Model).
React maintains two instances of the Virtual DOM: one representing the current state of the DOM and the other the state after the last update. This allows React to compare two versions of the Virtual DOM during the reconciliation process, identifying differences and minimizing the number of necessary updates in the real DOM. As a result, instead of performing costly operations on the real DOM with each change, updates are limited to absolutely necessary changes, resulting in faster rendering and better responsiveness of the application.
Understanding this process by the candidate not only indicates their ability to effectively manage application resources but also emphasizes a deep understanding of how React manages updates, which is crucial in developing more complex applications.
Question 3: What are the differences between class components and functional components in React?
As someone conducting job interviews, I pay attention to this question to assess whether the candidate is up to date with the evolution of practices in React. Currently, functional components, supported by hooks such as useState and useEffect, dominate in new React projects due to their simplicity and code readability. These tools enable efficient state management and component lifecycle handling without the need for more complex classes.
Example of a Functional Component with Hooks
The functional approach with hooks is currently the most popular method of building components in React. The following example shows how to efficiently manage state and side effects when fetching data from an external API:
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
const [isLoading, setIsLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
setData(data);
setIsLoading(false);
})
.catch(error => {
setError(error);
setIsLoading(false);
});
}, []);
if (isLoading) {
return <p>Loading...</p>;
}
if (error) {
return <p>Error: {error.message}</p>;
}
return <div>{data ? <p>Data fetched: {data.someField}</p> : <p>No data found.</p>}</div>;
}
export default DataFetcher;
Example of a Class Component
Although currently less common, understanding class components is still valuable, especially in the context of older applications. Here's how state management and side effects would be handled in a traditional class component:
import React, { Component } from 'react';
class DataFetcher extends Component {
constructor(props) {
super(props);
this.state = {
data: null,
isLoading: true,
error: null
};
}
componentDidMount() {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data: data, isLoading: false }))
.catch(error => this.setState({ error: error, isLoading: false }));
}
render() {
const { data, isLoading, error } = this.state;
if (isLoading) {
return <p>Loading...</p>;
}
if (error) {
return <p>Error: {error.message}</p>;
}
return <div>{data ? <p>Data fetched: {data.someField}</p> : <p>No data found.</p>}</div>;
}
}
export default DataFetcher;
Understanding the once-popular pattern of dividing components into presentational and container components is also valuable, especially in the context of older applications. Therefore, I expect candidates to be able to explain how state management and component lifecycles work in class components and how they differ from approaches used in functional components. Understanding these mechanisms not only demonstrates the candidate's technical proficiency but also their ability to adapt to different design paradigms and maintain code in older systems.
Question 4: How do you manage global application state in React?
Managing global state is a key aspect of building complex web applications in React. Therefore, it's worth preparing to discuss various methods and tools you can use to efficiently pass data between components.
Preparing for different approaches:
Redux:
Redux is one of the most popular solutions for state management, which applies principles such as single source of truth, immutability, and predictable data flow. It's worth familiarizing yourself with the basic concepts of Redux, such as stores, reducers, and actions, as well as understanding how these elements work together in managing application state.
Context API:
Introduced in React 16.3, the Context API makes it easier to pass data through the component tree without the need for prop drilling. Knowing this API can be particularly useful, as it's a less complicated solution than Redux and often sufficient in smaller applications or where state management isn't too complex.
MobX, Recoil, or others:
What projects have I worked on where I managed global state? What tools did I use and why?
This question will help you reflect on and organize your experiences related to state management in different projects. Preparing a specific answer where you describe your chosen solutions and justify their choice will demonstrate your practical skills and decision-making abilities in architectural context.
Question 5: What are your experiences with testing applications in React? What tools and methods do you use to ensure code quality?
Testing is an essential part of the application development process, especially in React, where components and state logic can become complex. Proper testing not only ensures that the application works as expected but also helps maintain code, its scalability, and refactoring.
Basic methods and tools:
Jest:
This is a popular testing tool in JavaScript, often used with React. It's ideal for writing unit tests and integration tests, offering strong support for mocking, timing, and assertions. It's worth knowing the basics of Jest, including how to configure the test environment, use matchers, or mock modules and functions.
Testing Library:
React Testing Library promotes an approach to testing that focuses on user interactions with components, which is closer to behavior-driven tests. Testing Library helps ensure that components not only work technically but are also functional from the user's perspective, which is crucial for building useful interfaces.
End-to-end testing (E2E):
Tools like Cypress or Selenium are used for end-to-end testing, which simulate real user scenarios, testing the entire application from front-end to back-end. Knowing these tools is important when you need to check how the entire system cooperates in performing typical user tasks.
Summary
Recruitment questions about React, such as those discussed above, aim not only to test candidates' theoretical knowledge but also to understand the deeper mechanisms of this library and the technical foundations of building applications. By asking questions about JSX, state management, component lifecycles, global state management, and testing, I assess how well candidates know and understand React and how effectively they can use this knowledge in practice.
Additionally, an important part of the recruitment process is the practical part, the so-called live coding. During this session, I observe how candidates approach coding, what practices they use, and how they deal with problems in real time. This allows me to assess not only the quality of the produced code but also the thinking process, problem-solving creativity, and ability to adapt under pressure, which is as important as theoretical knowledge.
The job interview focuses on these aspects to ensure that a potential employee will not only effectively work on existing projects but also contribute to the development and innovation within the team.