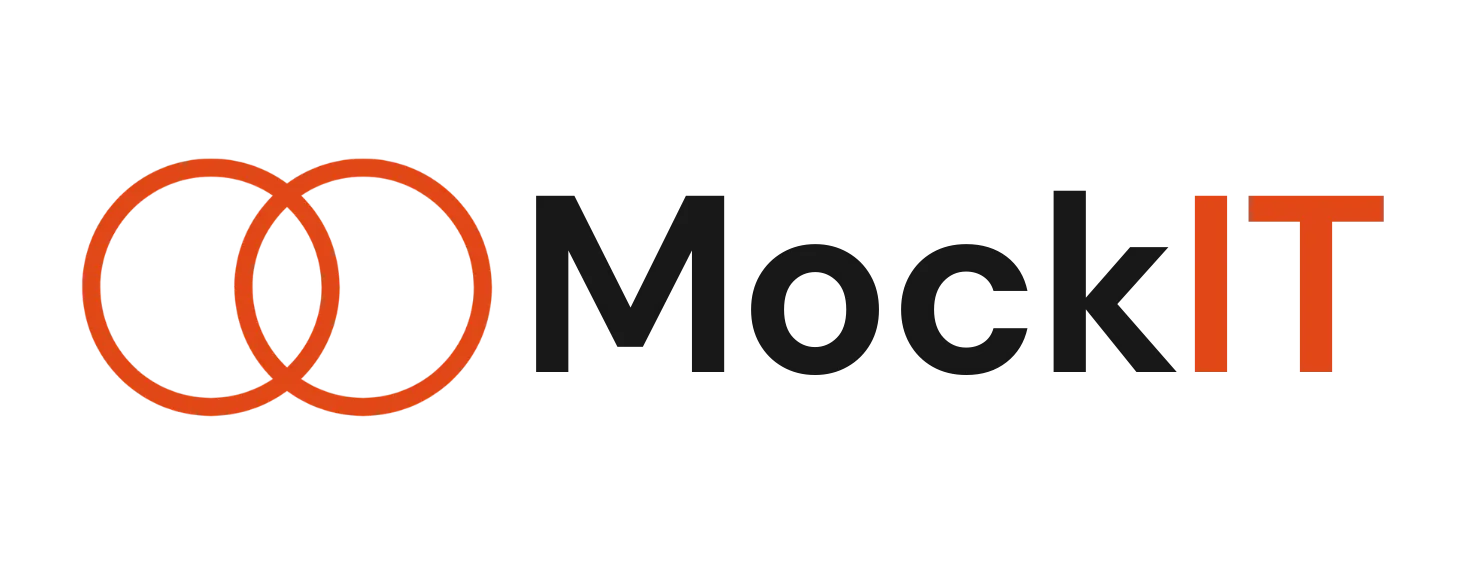
Do you get the feeling that no one is reading your CV? Not getting invitations to recruitment interviews?
Test your technical skills and let MockIT help find you a job in IT!
Advanced Questions for React Developer Interviews
Are you no longer a Junior and looking to change jobs? In this article, you'll learn more advanced interview questions related to React and Frontend Development.
Intro
In the previous article, we focused on basic interview questions that appear in interviews, such as discussing JSX, Redux, or the Context API.
The topics covered by these questions are essential to understanding the basic mechanisms and techniques used in everyday work with React. However, as the demand for more advanced and specialized skills grows, there is a need to delve into more complex aspects of this technology.
In today's article, we move on to advanced topics that are crucial not only for assessing candidates' seniority levels but also for understanding their in-depth knowledge of the React ecosystem.
We will discuss issues that require not only technical proficiency but also the ability to effectively manage complex projects and innovative thinking in application architecture. These advanced issues allow for assessing candidates' technical skills as well as their problem-solving abilities, designing scalable solutions, and adapting to rapidly changing technological environments.
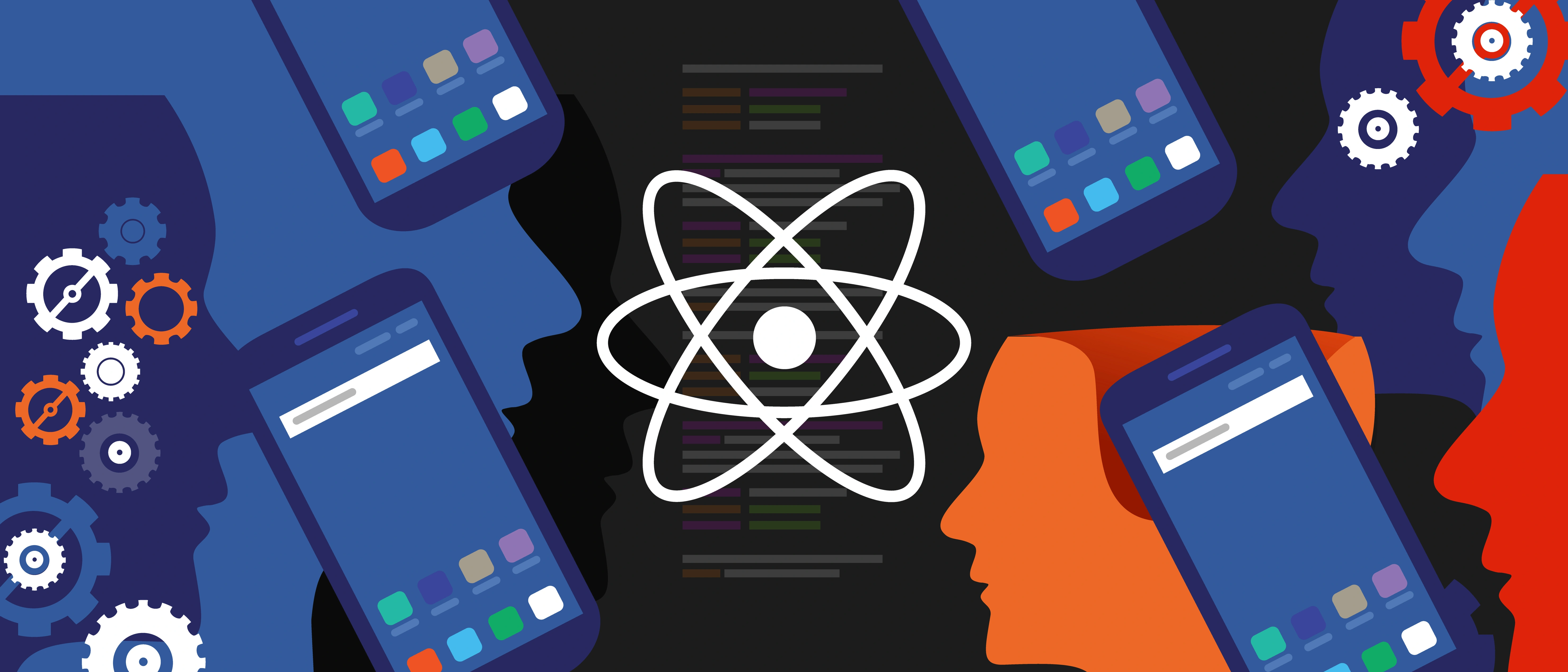
Differences Between MPA, SPA, SSR, and SSG Architectures
When designing web applications, developers can choose between different architectures, each with its specific advantages and limitations. Understanding the differences between multi-page architecture (MPA), single-page application (SPA), server-side rendering (SSR), and static site generation (SSG) is crucial for selecting the appropriate approach for a particular project.
MPA (Multi-Page Application)
MPA is a traditional type of web application where each page modification results in loading a new page from the server. This approach is well-known from the early internet and is still popular in applications that require strong separation between individual pages, such as corporate portals or e-commerce platforms. The advantages of MPA include simplicity in scaling and SEO optimization, as each page is indexed by search engines as a separate entity.
SPA (Single Page Application)
SPA are applications that load the entire application at the start and then dynamically update the content using JavaScript (in the browser) without reloading the entire page. Users experience smoother transitions and faster application responses because most resources, including HTML, CSS, and scripts, are loaded only once at the beginning. SPA is often used in applications requiring interactivity and quick response, such as dashboards or analytical tools. A high degree of interactivity is ensured through browser-side rendering. The downside can be initial loading performance and potential SEO issues, which can be overcome with proper optimization techniques and tools like prerendering.
SSR (Server-Side Rendering)
SSR is a technique where page content is generated on the server side for each user request. The page is quickly available to the user, which is beneficial for SEO and initial loading time, as search engines can index the content more efficiently. After loading the page, a hydration process occurs where JavaScript scripts are fetched, enabling dynamic interactions similar to SPA. SSR is often used in modern frameworks like Next.js, which can automatically manage server-side and client-side rendering.
SSG (Static Site Generation)
SSG involves generating static HTML pages at build time. Each page is pre-built and ready for quick serving, which is ideal for sites where the content changes rarely but needs to be very fast. After the initial load, similar to SSR, a hydration process can occur where JavaScript scripts are fetched, allowing for dynamic interactions without the need to refresh the page. SSG is often used in blogs, product documentation, and portfolio websites where SEO and loading speed are critical.
What Strategies and Techniques Do You Use to Optimize the Performance of React Applications?
In the development process, performance optimization plays a key role in ensuring smooth user interaction and efficient resource utilization. However, just as important as knowing optimization techniques is the ability to take a balanced approach to optimization.
In the context of job interviews, I value candidates who understand that 'premature optimization' can be as harmful as no optimization at all. Asking about optimization not only assesses a candidate's technical knowledge but also their decision-making in effective resource management and recognizing when optimization is appropriate.
Lazy Loading Components
Lazy loading components is a technique that allows loading code only when it is needed. This can significantly reduce the initial loading time of the application, which is especially beneficial in large projects with many different functionalities that are not needed immediately at startup.
Using React.lazy() and Suspense
React.lazy() allows defining a component that will be dynamically loaded, and Suspense enables specifying what should be displayed while that component is loading (e.g., a loading indicator). Below are examples illustrating how these functions can be used.
Example:
Suppose we have a HeavyComponent that is large and rarely used, so it is ideal for lazy loading.
// HeavyComponent.js
import React from 'react';
const HeavyComponent = () => {
return <div>Heavy Component here</div>;
};
export default HeavyComponent;
To implement lazy loading for this component, we can use React.lazy to dynamically import:
// App.js
import React, { Suspense } from 'react';
const HeavyComponent = React.lazy(() => import('./HeavyComponent'));
function App() {
return (
<div>
<h1>Hello World!</h1>
<Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</Suspense>
</div>
);
}
export default App;
In the example above, the HeavyComponent is only loaded when necessary, i.e. when the user is on the right path or in the right part of the application. Suspense ensures that instead of the component, users will see the message 'Loading...' until the HeavyComponent is loaded and ready to render.
Benefits of Lazy Loading
Reduced Loading Time: By not loading all components at once, we can reduce the initial loading time of the application.
Resource Management: Lazy loading allows for better resource management, loading only those components that are currently needed.
Performance Improvement: Users experience faster application interaction times because they don't have to wait for the entire application to load at once.
Memoizing Components and Function Results
Memoization is an optimization technique that involves remembering the results of function calls or component rendering to avoid expensive operations during subsequent renderings. In React, memoization helps minimize the number of re-renders by storing results and reusing them when input data hasn't changed.
React.memo()
React.memo is a higher-order component (HOC) that wraps a functional component and prevents unnecessary re-rendering if props haven't changed. It is useful when dealing with components that receive complex objects or large amounts of data as props.
Example:
const MyComponent = React.memo(function MyComponent(props) {
/* render using props */
return <div>{props.children}</div>;
});
useMemo()
The useMemo hook allows for memoizing values. This is useful when a value is expensive to compute, and we don't want it to be recomputed on every render unless its dependencies change.
Example:
import React, { useMemo } from 'react';
function ExpensiveComponent({ count }) {
const expensiveValue = useMemo(() => {
return computeExpensiveValue(count);
}, [count]);
return <div>{expensiveValue}</div>;
}
function computeExpensiveValue(num) {
console.log('Computing expensive value');
return num * 2; // Simulate heavy operation
}
useCallback()
useCallback is a hook that returns a memoized version of the passed callback function that only changes when its dependencies change. This is particularly useful in cases where passing callbacks can lead to unnecessary renders in child components.
Example:
import React, { useCallback, useState } from 'react';
function MyButton() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount((c) => c + 1);
}, []); // Dependencies are empty so function won't update
return <button onClick={increment}>Count: {count}</button>;
}
Using useMemo and useCallback allows control over expensive operations and functions, minimizing unnecessary re-renders. It's worth noting that excessive use of these hooks can lead to additional overhead, so they should be used only when there are clear benefits from optimization.
Optimizing List and Element Rendering
When rendering large lists or tables, performance can be significantly improved by limiting the number of element re-renders. Using React.memo in conjunction with proper keying of elements (using unique and stable values as keys) can help with optimization. Additionally, tools like react-window or react-virtualized allow rendering only the elements visible to the user, reducing the DOM load.
Optimal State Management
Managing state in large React applications can impact performance, especially when state updates cause unnecessary re-renders. Using context, Redux, or other state managers carefully and minimizing the number of components connected to global state can reduce overhead. Additionally, applying techniques like debouncing or throttling on event handlers can limit the number of state updates per second.
Profiling and optimization based on real metrics
React Developer Tools offer a panel for profiling component performance, helping identify which components are rendered most frequently and how long their renderings take. Analyzing this data allows pinpointing bottlenecks and making appropriate code modifications for more effective optimization.
What are the benefits of using TypeScript in a React project and what challenges may arise when integrating TypeScript with an existing React project?
By asking this question as a recruiter, I aim not only to assess candidates' technical knowledge of TypeScript but also to understand how comfortable they are with its application in diverse project contexts. I'm also interested in the candidate's approach to decision-making regarding TypeScript adoption for legacy projects, where integration may present various challenges. This question provides deeper insight into how candidates weigh the pros and cons of TypeScript usage and how they plan and manage technological migration in real project conditions.
Benefits of using TypeScript in React
Improved code quality: TypeScript introduces static typing, which can significantly improve code quality by detecting type errors early and providing clearer definitions of interfaces and types.
Facilitates refactoring: Due to static typing, TypeScript facilitates safe code refactoring because type changes propagate throughout the code, minimizing the risk of introducing errors.
Better team collaboration: Types in TypeScript can serve as documentation, which is particularly useful in larger teams where developers may not be familiar with the entire application codebase. Creating typed code provides excellent future documentation, easing new team members' understanding and integration with the project.
Increased developer productivity: Features such as code autocompletion and immediate feedback on errors contribute to faster and more efficient code development.
Challenges in integrating TypeScript with an existing React project
Learning curve: For teams unfamiliar with TypeScript, the initial learning curve can be challenging and may lead to a short-term decrease in productivity.
Code migration: Transforming an existing JavaScript codebase to TypeScript can be time-consuming and error-prone, especially in large projects.
Managing types for external libraries: Not all JS libraries have good TypeScript type support, which can add work in creating or managing custom type definitions.
Increased tooling complexity: Introducing TypeScript to a project often involves updating or modifying the existing stack of developer tools, which can introduce additional complexity.
Summary
The questions discussed in this article serve not only as a tool to assess candidates' technical proficiency for the Front-end Developer position but also as a way to understand their ability in making architectural decisions and supporting technical leaders. By asking questions about advanced topics such as performance optimization, TypeScript integration, or differences between application architectures, we can assess how candidates think strategically and how their work can contribute to the long-term success of the project.
These questions not only help determine if a candidate possesses the necessary technical skills but also if they are capable of working in a team where technical decisions directly impact product development, scalability, and maintenance. Therefore, evaluating such skills is crucial in the selection process, especially for roles that require not only daily coding but also strategic thinking and the ability to adapt to rapidly changing technological and business requirements.