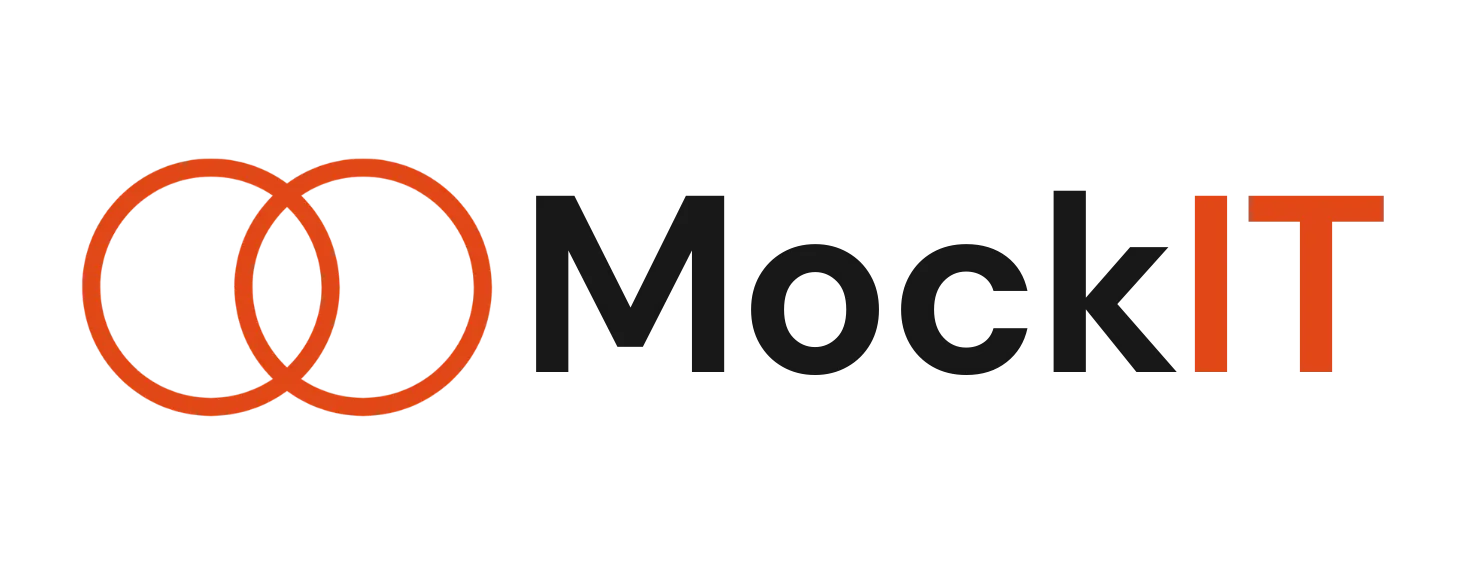
Do you get the feeling that no one is reading your CV? Not getting invitations to recruitment interviews?
Test your technical skills and let MockIT help find you a job in IT!
Python Developer - job interview questions about lists + answers
If you are just starting your Python programming career, this article is for you! It describes the most basic questions about lists in Python. An absolute must-have for every Junior Python Developer.
Intro
In the world of programming, knowledge of data structures is crucial for effective software development. Among the many useful tools that Python offers, lists are one of the fundamental elements. They are versatile, flexible, and widely used by both beginner and experienced Python programmers.
During the recruitment process, questions about data structures are standard in many programming languages, including popular ones like JavaScript and Java. They quickly determine the candidate's level of expertise - not only during the technical question section but also during the recruitment tasks.
This article was created so you can learn about sample questions regarding lists in Python along with answers. Due to the level of difficulty, these questions most often appear during interviews for the position of Junior Python Developer.
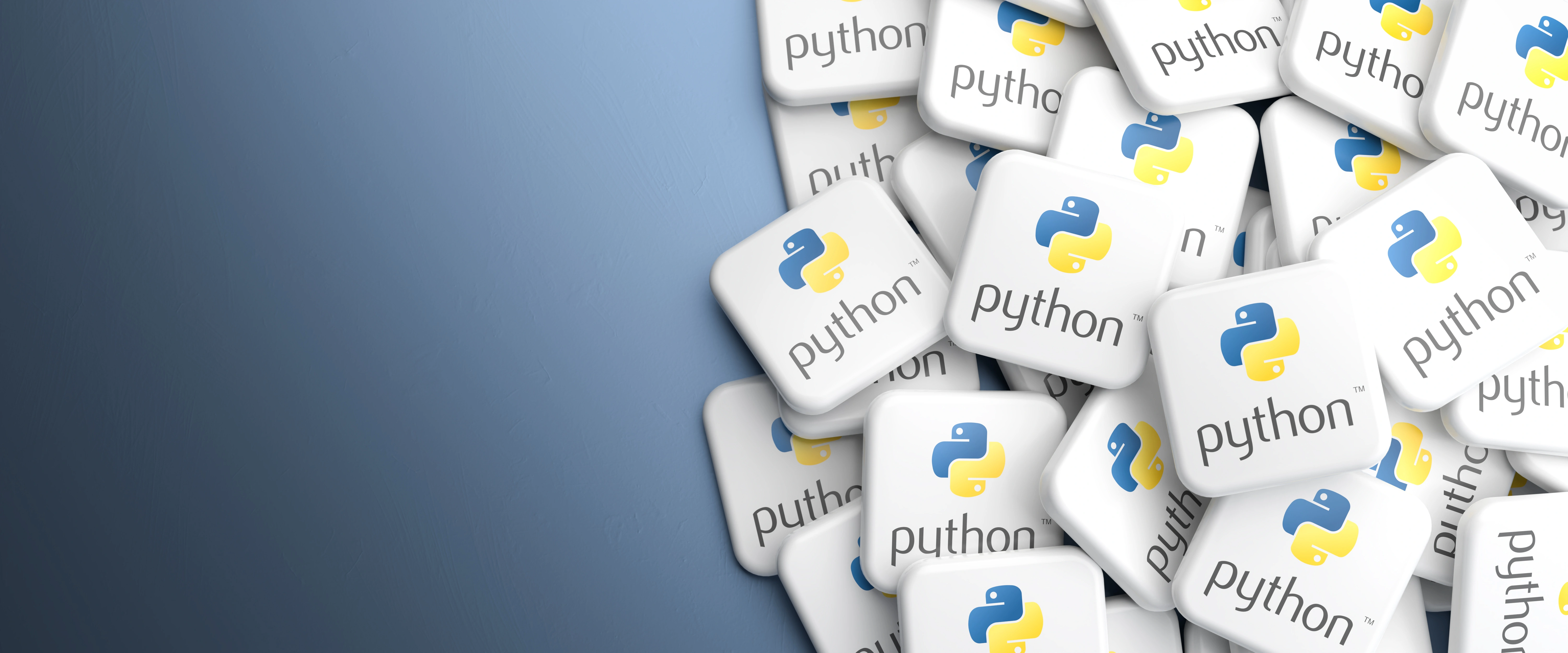
What are the main features of lists in Python?
A list in Python is a basic, dynamic data structure. It stores a collection of elements and maintains their order.
Dynamic size
Lists in Python can dynamically change their size. We can add new elements to the list using the append(), extend(), or insert() methods, and we can also remove elements using the remove(), pop() methods, or the del operator.
Heterogeneity of elements
Unlike many other programming languages, lists in Python can store elements of different data types. This means that in one list, we can have integers, floating-point numbers, strings, and even other lists.
Indexing and access
Lists in Python support indexing, which allows access to individual elements by their indices. Indexing starts from zero. It is also possible to use negative indices, which refer to elements from the end of the list.
Mutability
Lists are mutable, meaning we can change their contents after creation. We can change the values of individual elements, as well as add or remove elements.
names = ['Michał', 'Kinga', 'Monika']
names[2] = 'Boromir'
# Result: ['Michał', 'Kinga', 'Boromir']
What are the differences between the append(), extend(), and insert() methods for lists in Python?
Among the many methods available for lists, three – append(), extend(), and insert() – are often used to add new elements. Although they may seem similar at first glance, each serves a different purpose and works in a specific way. Let's take a closer look at these methods to understand how they differ and how to use them correctly.
The append() method
The append() method is used to add a single element at the end of the list. It is a simple way to extend a list with a single object.
The extend() method
The extend() method allows you to add multiple elements to the list at once. It takes an iterable object (e.g., another list) as an argument and adds each of its elements to the end of the original list.
example_list = [1, 2, 3]
example_list.extend([4, 5, 6])
# Result: [1, 2, 3, 4, 5, 6]
The insert() method
The insert() method allows you to insert an element at any position in the list. It requires two arguments: the index at which the element should be added and the element itself.
example_list = [1, 2, 3]
example_list.insert(1, 'a')
# Result: [1, 'a', 2, 3]
How to sort a list in Python? Is there a difference between the sort() method and the sorted() function?
Sorting lists is one of the frequently performed operations in programming. Python offers two main ways to sort lists: the sort() method and the sorted() function. Although both methods serve the same purpose, they work in slightly different ways. Understanding these differences will help you make the right choice depending on the specific situation.
The sort() method
The sort() method is a built-in list method in Python that sorts the elements of the list in place, meaning it modifies the original list. By default, it sorts in ascending order, but you can use optional arguments to change its behavior.
example_list = [3, 1, 4, 1, 5, 9, 2]
example_list.sort()
# Result: [1, 1, 2, 3, 4, 5, 9]
In the example above, the sort() method sorts the list lista in place. The original list is modified. Optional arguments:
reverse: If True, the list is sorted in descending order.
key: A function that serves as a key for comparing elements.
example_list = ["apple", "banana", "cherry"]
example_list.sort(key=len)
# Result: ['apple', 'cherry', 'banana']
The sorted() function
The sorted() function sorts the elements of any iterable object (such as lists, tuples, strings) and returns a new list containing the sorted elements. The original object remains unchanged.
example_list = [3, 1, 4, 1, 5, 9, 2]
new_example_list = sorted(example_list)
print(new_example_list) # Output: [1, 1, 2, 3, 4, 5, 9]
print(example_list) # Output: [3, 1, 4, 1, 5, 9, 2]
Like the sort() method, the sorted() function also accepts the key and reverse arguments.
example_list = ["apple", "banana", "cherry"]
new_example_list = sorted(example_list, key=len, reverse=True)
print(new_example_list) # Output: ['banana', 'cherry', 'apple']
Summary
sort(): A list method that sorts the original list in place. It does not return a new list but modifies the existing one. Used mainly when you do not need to keep the original version of the list.
sorted(): A built-in function that returns a new sorted list from any iterable object, leaving the original object unchanged. Ideal when you want to keep the original object.
Knowing these differences allows you to better tailor sorting methods to specific needs in your code. The choice between sort() and sorted() depends on whether you want to modify the existing list or prefer to work with a copy of the list.
How to get a list with unique values?
In Python, it is often necessary to obtain a list containing only unique values from the original list. There are several ways to achieve this using different tools and methods available in Python. Let's look at some of the most commonly used techniques.
Using a set
The simplest and most commonly used way to get unique values from a list is to use a set. Sets in Python automatically eliminate duplicates because they can only contain unique elements.
example_list = [1, 2, 2, 3, 4, 4, 5]
uniques = list(set(example_list))
# Result: [1, 2, 3, 4, 5]
Using a loop and an auxiliary list
Another way is to use a loop to iterate through the original list and add elements to a new list only if they are not already present.
example_list = [1, 2, 2, 3, 4, 4, 5]
uniques = []
for element in example_list:
if element not in uniques:
uniques.append(element)
# Result: [1, 2, 3, 4, 5]
Besides the two mentioned, there are, of course, other ways, e.g., using the pandas library or list comprehension.
How to get a sublist from a list? Explain the use of the slicing operator.
In Python, getting a sublist from an existing list is very simple using the slicing operator. This operator allows you to select a specific range of elements from a list in a clear and intuitive way. Understanding the slicing mechanism is crucial for effective list manipulation.
Basic slicing syntax
lista[start:stop:step]
start: The index at which the slice starts (inclusive).
stop: The index at which the slice ends (exclusive).
step: Step, which determines the stride between elements.
example_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
sub_example_list = example_list[1:8:2]
print(sub_example_list) # Output: [1, 3, 5, 7]
Slices can also take negative values for start, stop, and step. Negative values refer to indices from the end of the list.
example_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
sub_example_list = example_list[-5:-1]
print(sub_example_list) # Output: [5, 6, 7, 8]
sub_example_list = example_list[: -1]
print(sub_example_list) # Output: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
List comprehension - what is it and how to use it?
List comprehension is an advanced and elegant construct in Python that allows you to create new lists from existing iterable objects in a concise and readable way. Using list comprehension enables combining loops and logical conditions in a single, compact expression.
Basic list comprehension syntax
new_list = [expression for element in iterable]
expression: An expression that calculates values for the new list.
element: A variable that holds the current element from the iterable.
iterable: An iterable object (e.g., list, tuple, set) from which elements are taken.
numbers = [1, 2, 3, 4, 5]
quadratics = [x**2 for x in numbers]
print(quadratics) # Output: [1, 4, 9, 16, 25]
We can also add conditions to list comprehensions to filter elements to be included in the new list.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even = [x for x in numbers if x % 2 0]
print(even) # Output: [2, 4, 6, 8, 10]
In this case, only even numbers from the list numbers are added to the new list.
How to use the built-in filter() function?
The built-in filter() function in Python is used to filter elements from a sequence based on a specified condition. It takes two arguments: a filtering function and the sequence to be filtered.
The general syntax of the filter() function is as follows: filter(filtering_function, sequence)
The filter() function returns an iterator object that contains only those elements from the sequence for which the filtering function returns True. To get the result as a list, you can use the list() function to convert the iterator object to a list. This is standard practice. The filter() function is often used in conjunction with lambda anonymous functions.
number = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even = list(filter(lambda x: x % 2 0, number))
print(even) # Output: [2, 4, 6, 8, 10]
How to use the built-in map() function?
The built-in map() function in Python is used to transform elements of a sequence using a specified function. It takes two arguments: a mapping function and the sequence to be transformed.
The map() function returns an iterator object that contains the results of applying the mapping function to each element of the sequence. To get the result as a list, you can use the list() function to convert the iterator object to a list. Similar to the filter() function, this is standard practice.
What are the differences between deepcopy and copy on nested lists?
In Python, objects can be copied in several ways, depending on the depth of copying. For simple, flat lists, a shallow copy is sufficient, but for nested lists, a deep copy is often necessary. Understanding the differences between copy and deepcopy is crucial for proper data management in such structures.
Shallow Copy
The copy() function creates a shallow copy of the object. For lists, this means a new list is created with references to the same elements as the original list. This means that changes in nested elements will be visible in both the original and the copy.
import copy
example_list = [[1, 2, 3], [4, 5, 6]]
shallow_copy = copy.copy(example_list)
# Modifying inner element
shallow_copy[0][0] = 'a'
print(example_list) # Output: [['a', 2, 3], [4, 5, 6]]
print(shallow_copy) # Output: [['a', 2, 3], [4, 5, 6]]
Deep Copy
The deepcopy() function creates a deep copy of the object. This means that all nested objects are recursively copied, creating a fully independent copy of the original structure. Changes in nested elements will not affect the original object.
import copy
example_list = [[1, 2, 3], [4, 5, 6]]
deep_copy = copy.deepcopy(example_list)
# Modyfikacja zagnieżdżonego elementu
deep_copy[0][0] = 'a'
print(example_list) # Output: [[1, 2, 3], [4, 5, 6]]
print(deep_copy) # Output: [['a', 2, 3], [4, 5, 6]]
Summary
Knowledge of lists in Python is crucial for any Python programmer, regardless of experience. Familiarizing yourself with the topics presented in this article will not only help you better prepare for interviews for Python Developer positions but also deepen your knowledge.
Remember that the key to success is not only theoretical knowledge but also the ability to apply it in practice. This can also be tested in a recruitment interview. Regularly practicing tasks and solving problems will help you gain confidence and better showcase your skills during the interview.