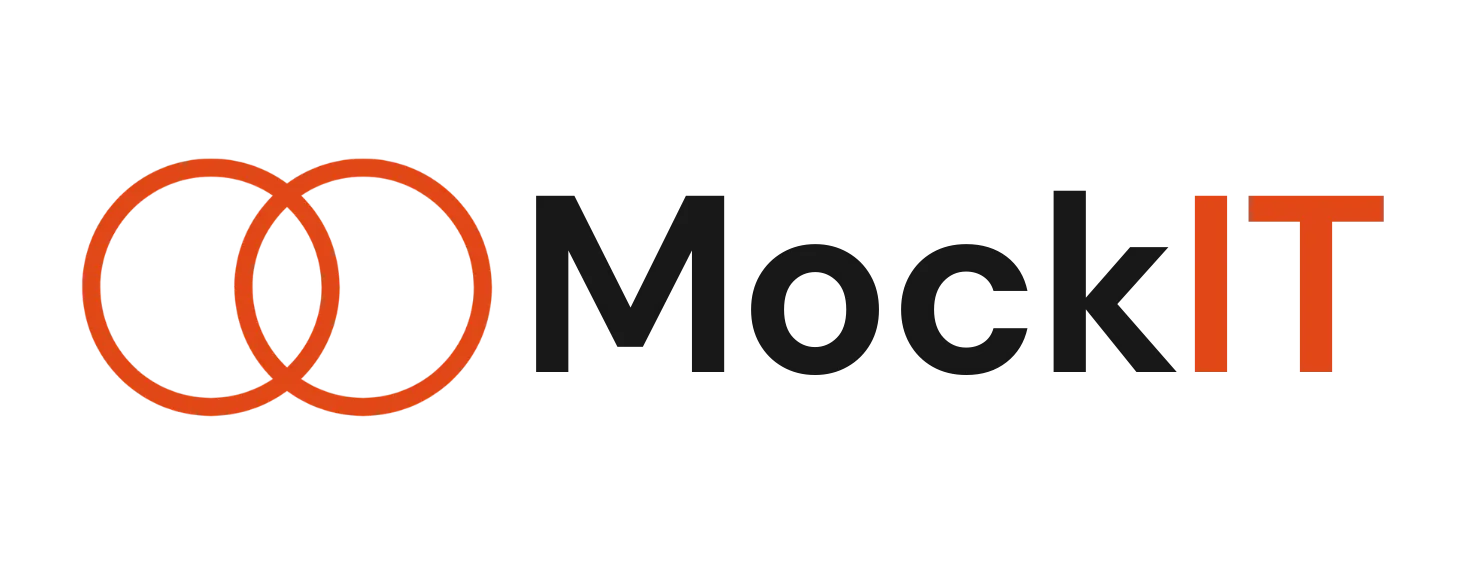
Do you get the feeling that no one is reading your CV? Not getting invitations to recruitment interviews?
Test your technical skills and let MockIT help find you a job in IT!
Java Interview Questions and Answers - Essential Knowledge for Java Developers
Are you preparing for a Java job interview? If so, you're in the right place - today we'll present you with a collection of topics that every candidate preparing for a Mid or Junior level interview should review.
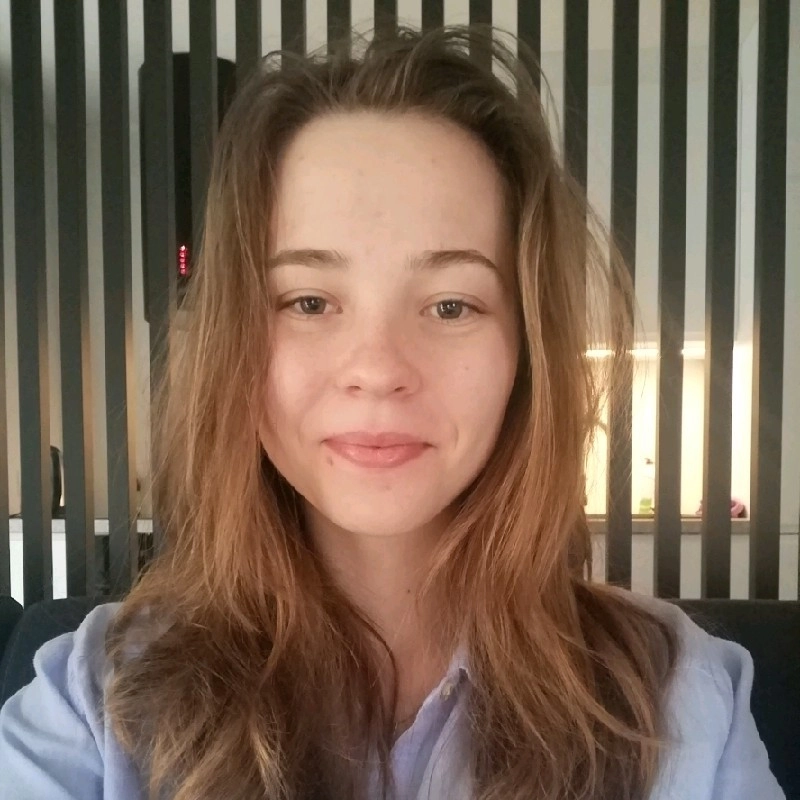
Intro
I can assure you that a solid understanding of Java basics is your ticket to a successful interview. The recruiter will be able to ask increasingly difficult questions, and it's correct and comprehensive answers to these questions that will allow you to stand out among the crowd of candidates.
You can treat the collection of topics presented here as a checklist to review before every interview for a Java Developer position. The list includes only Java topics - Spring, Hibernate, SQL, git, and sample interview questions along with answers will be discussed in a separate article.
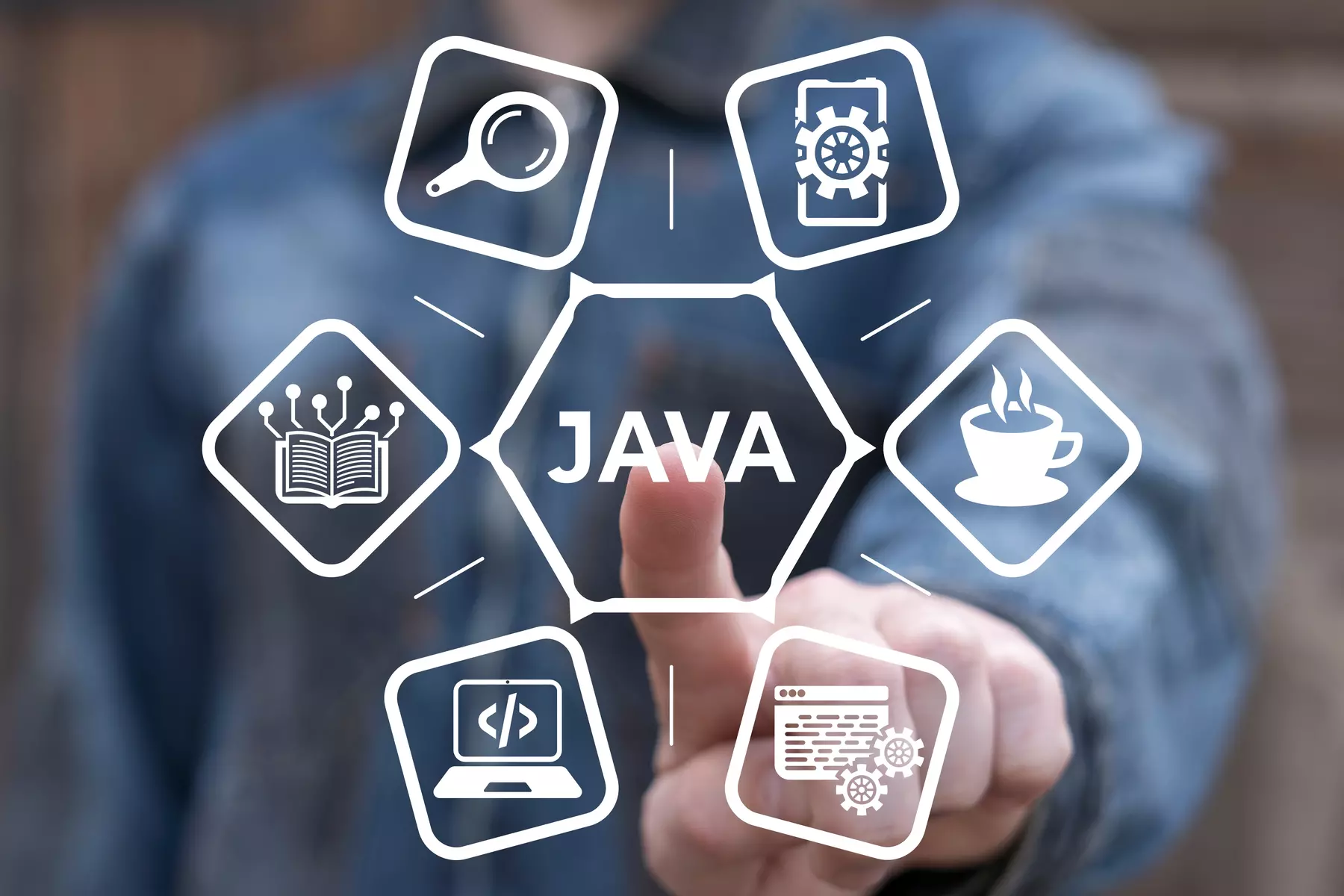
Junior Java Developer Job Interview - List of Topics to Review
Java Core
Let's start with some warm-up - basic concepts of the Java language. There are many of them, however, in the context of recruitment, the most important ones are:
object-oriented programming (discussed below),
overloading and overriding methods,
exceptions (discussed later)
pass-by-value and pass-by-reference,
primitive and non-primitive data types (constructors, Autoboxing and Unboxing),
final keyword (can we declare a final constructor?),
variables (static, global, local)
Java Collection
Without a good understanding of collections, there's no point in even starting the recruitment process - after all, storing and manipulating groups of objects is a fundamental task in java programming.
From total must-haves, lists, sets, maps (which theoretically do not implement the Collection interface, but belong to the Java Collection Framework) are certainly included, but it also wouldn't hurt to remind yourself what queues are, what computational complexities are, and for what purposes it's best to use individual collections.
Hash-based Collections
This topic definitely deserves a separate subpoint. Hash collections are those that use hashing functions for efficient searching and manipulation of elements. In addition to knowing the most popular collections like HashMap or HashSet, you need to remember about the hashCode and equals methods, as well as the contract between them.
It will certainly be useful to remind yourself how the HashMap implementation looks like and what collisions or the 'losing' effect of objects in HashMap are.
Exceptions
Questions about exceptions come up very often - after all, working with them is commonplace for every programmer, so even at the Junior level, you must be proficient in this topic. You probably don't need reminding about knowledge of checked and unchecked exceptions and handling exceptions using try or throws, but don't be surprised by more difficult questions, such as exceptions in lambda expressions.
Concurrency
A topic that not every developer encounters on a daily basis, but it's popular in recruitment interviews. Concurrency opens your eyes to many aspects of software development, so it's worth getting familiar with it, regardless of whether you have daily contact with it or not. To start with, read about: thread deadlock and starvation, race conditions, and how concurrency is supported in Java - synchronized, thread, volatile.
Object-Oriented Programming
Knowledge of object-oriented programming is crucial - after all, Java is an object-oriented language. You should be able to list object-oriented paradigms with your eyes closed, understand what SOLID is, and know the difference between an interface and an abstract class in java. It's also essential to know about inheritance, method overriding, variable declaration, and what access modifiers are in Java.
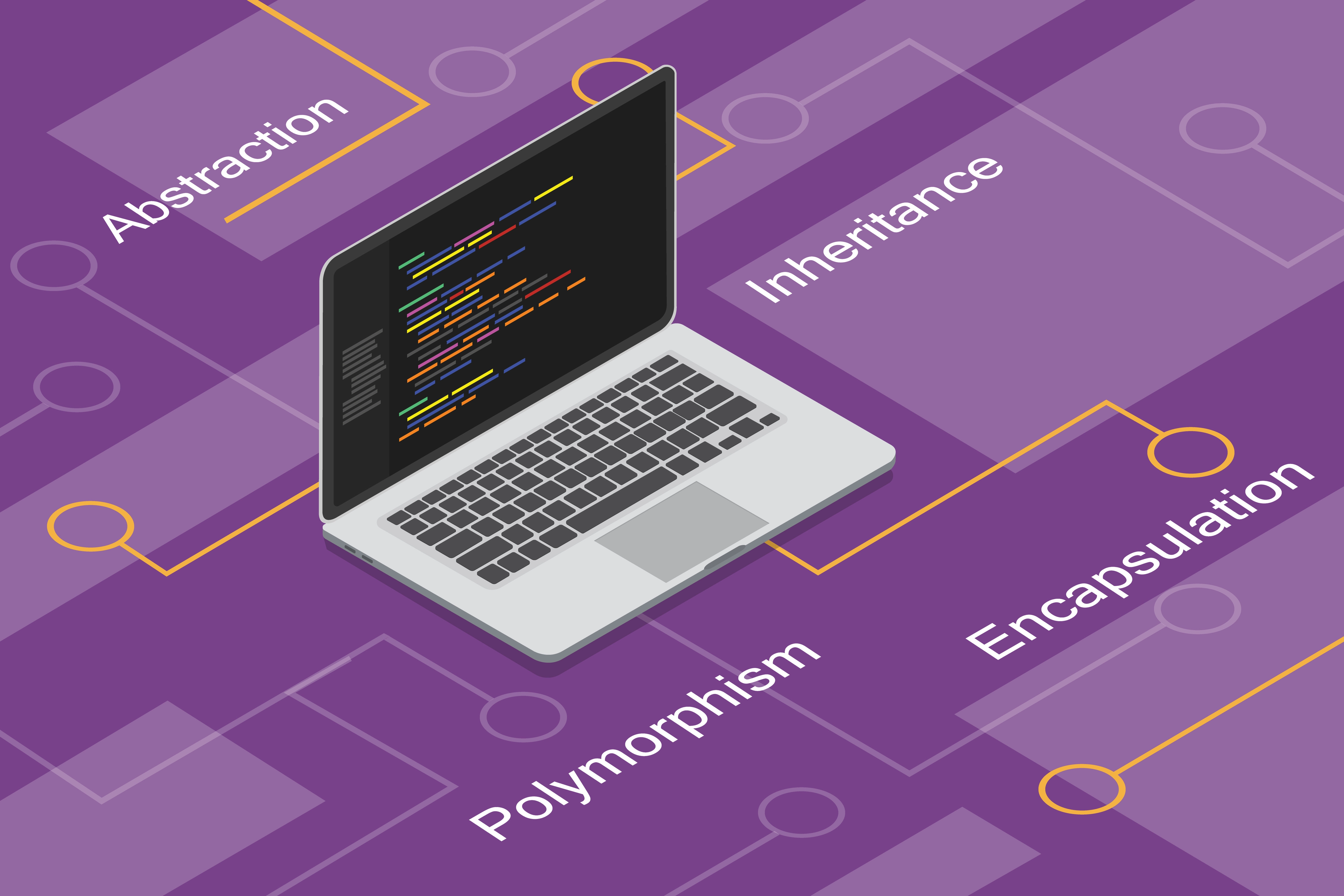
Immutable Objects
Questions about immutable objects often appear in interviews, and the question of how to create an immutable object is one of the more popular ones. In addition to answering these questions, don't forget the basics like when and why we use such objects.
Memory Management and Garbage Collector
Knowledge of these topics is essential for conscious work with the JVM (java virtual machine). Unfortunately, in these topics, you can expect questions from a very wide range from the recruiter. From must-haves, you should know what the stack, heap, and its divisions are, types of Garbage Collectors, and algorithms used by the Garbage Collector (these last two topics already touch on the mid-level).
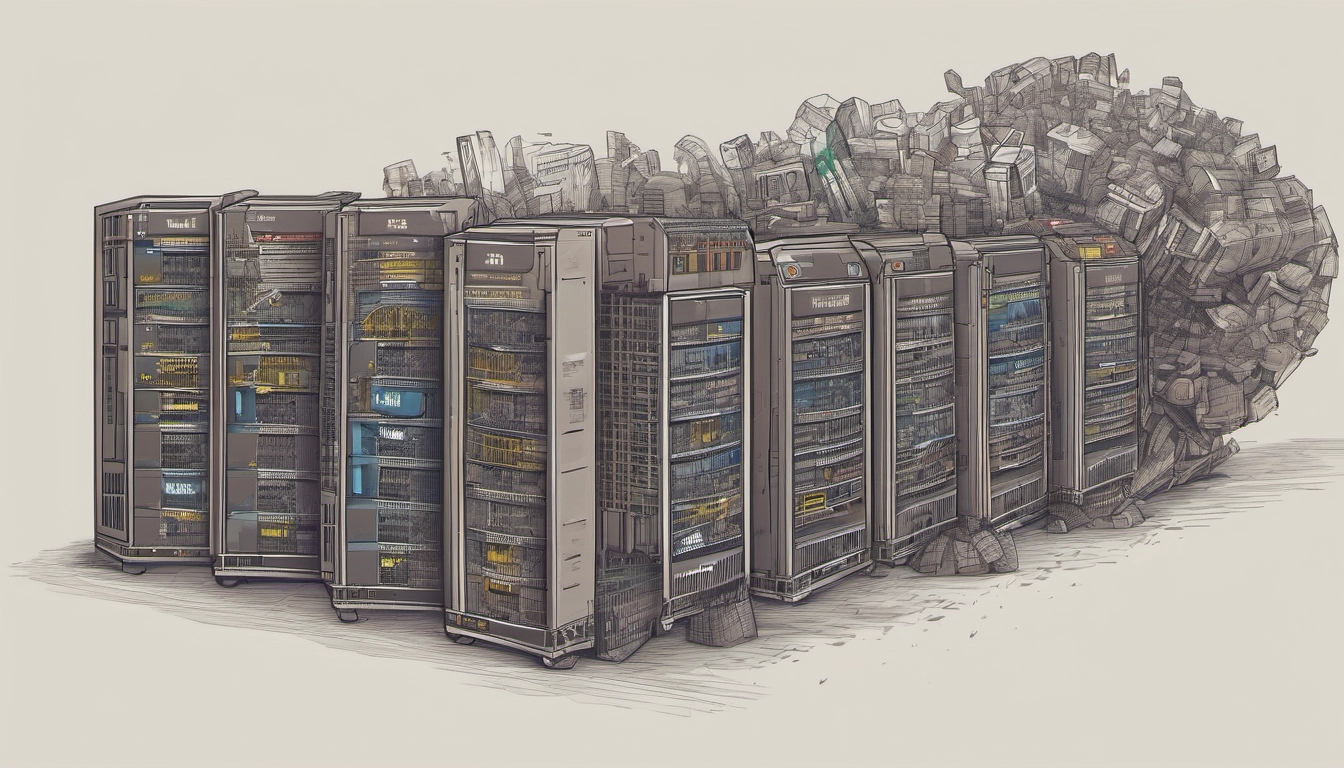
Design Patterns
The topic of design patterns is very extensive and generally not only related to Java. At the junior level, it's worth familiarizing yourself with the division of patterns into groups and getting acquainted with at least a few of the most popular patterns such as singleton, builder, factory, or strategy.
It's worth considering the fundamental question: why do we learn design patterns at all? The answer to this question is simple - to be able to apply proven and efficient solutions to recurring problems that are understandable to all programmers.
Java Stream API
A topic present since java 8, and every Java programmer should be fluent in it. It's certainly worth knowing the groups of operations on streams and knowing at least a few methods from each of them. From myself, I'll add that many people only associate two main groups of stream operations - terminal and intermediate, but few know that within intermediate operations, there are two more subgroups - stateless and stateful. Preparing with the stream API will not only help you answer recruiter questions, but who knows, maybe you'll also use this knowledge during live coding?
Functional Interfaces and Lambda Expressions
Although this topic is not as popular in interviews as collections or concurrency, ignorance of these concepts may result in a negative assessment from the recruiter. It's worth familiarizing yourself with at least a few basic functional interfaces (e.g. Function, Predicate, or Supplier) and what lambda expressions are and what they are used for.
Testing
Writing tests allows us to create reliable software, so they are a constant element of programmers' work. You definitely need to know how to create good tests, why we write them at all, how integration tests differ from unit tests, and what the test pyramid is - these are absolute basics. If you want to impress the recruiter with your knowledge, check out what TDD is and what its benefits are.
JRE, JVM, JDK
As a knowledgeable Java programmer, you should be able to distinguish them well and know what they are used for. Although knowledge of them is not usually used in everyday work, these are basic concepts related to the Java language.
Additionally, you can remind yourself what machine language (bytecode) is and how it happens that an java application compiled for one platform can be run smoothly on other operating systems and hardware platforms, and how to compile and execute Java code.
Java String
The topic of Strings in Java likes to appear in recruitments. It's not necessarily a must-have, but ignorance of them will certainly work to your disadvantage. It's worth reminding yourself how to create Strings, what String pool is, what the differences between String, StringBuffer, and StringBuilder are, and how we can compare Strings.
Are These Topics All You Need to Know Before a Job Interview?
The topics listed above are just the basics, and a lack of knowledge in these areas, even with knowledge of more advanced topics, can result in a negative assessment from the recruiter. Remember that besides theoretical knowledge, the candidate's ability to write good code is required.
The recruiter will assess the quality of your software by looking at your projects on GitHub and through practical recruitment tasks. Remember that it doesn't have to be just about implementing a specific task. Sometimes the recruiter can present you with existing code and ask you to point out errors in it or simply discuss it with you.
Don't forget to prepare to discuss your projects and experiences.
What Do I Need to Know When Preparing for a Mid Java Developer Interview?
If you're preparing for a Mid-level position interview, remember that your knowledge of the presented topics should be extensive - the recruiter will expect greater accuracy and a more in-depth understanding of the topics from you. You must answer the questions confidently and extensively.
For comparison of levels, I'll use the example of a quite popular question in interviews - what are synchronized and concurrent collections. If at the Junior level, the candidate has only partial knowledge of these collections (e.g., knows what they are but doesn't know implementation details), it will most likely be assessed neutrally. However, if at the Mid-level, the candidate does not know the characteristics of these collections, it may already have a negative impact on the assessment.
How to Achieve Success?
To succeed in an interview for a Junior or Mid Java Developer position, it's crucial not only to have a deep understanding of the Java language and related technologies but also the ability to apply them practically. Therefore, besides reviewing the mentioned topics, regular coding practice and solving technical problems are necessary. Also, don't forget to prepare for discussions about your projects and experiences that may interest the recruiter.
Remember that your passion for programming and willingness to develop are as important as the technical aspects of your knowledge. If you want to have a practice job interview in Java, get a certificate, and additionally get into the database provided to employers click here.
Good luck!